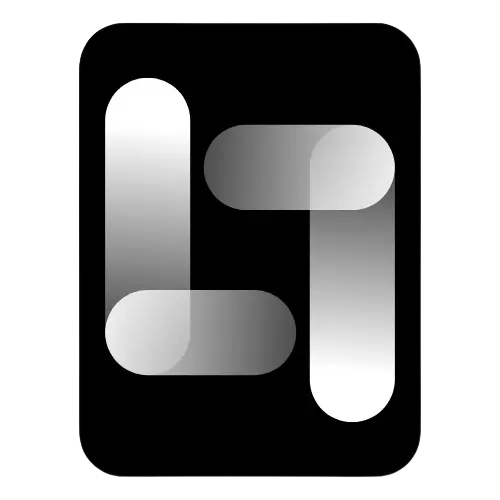
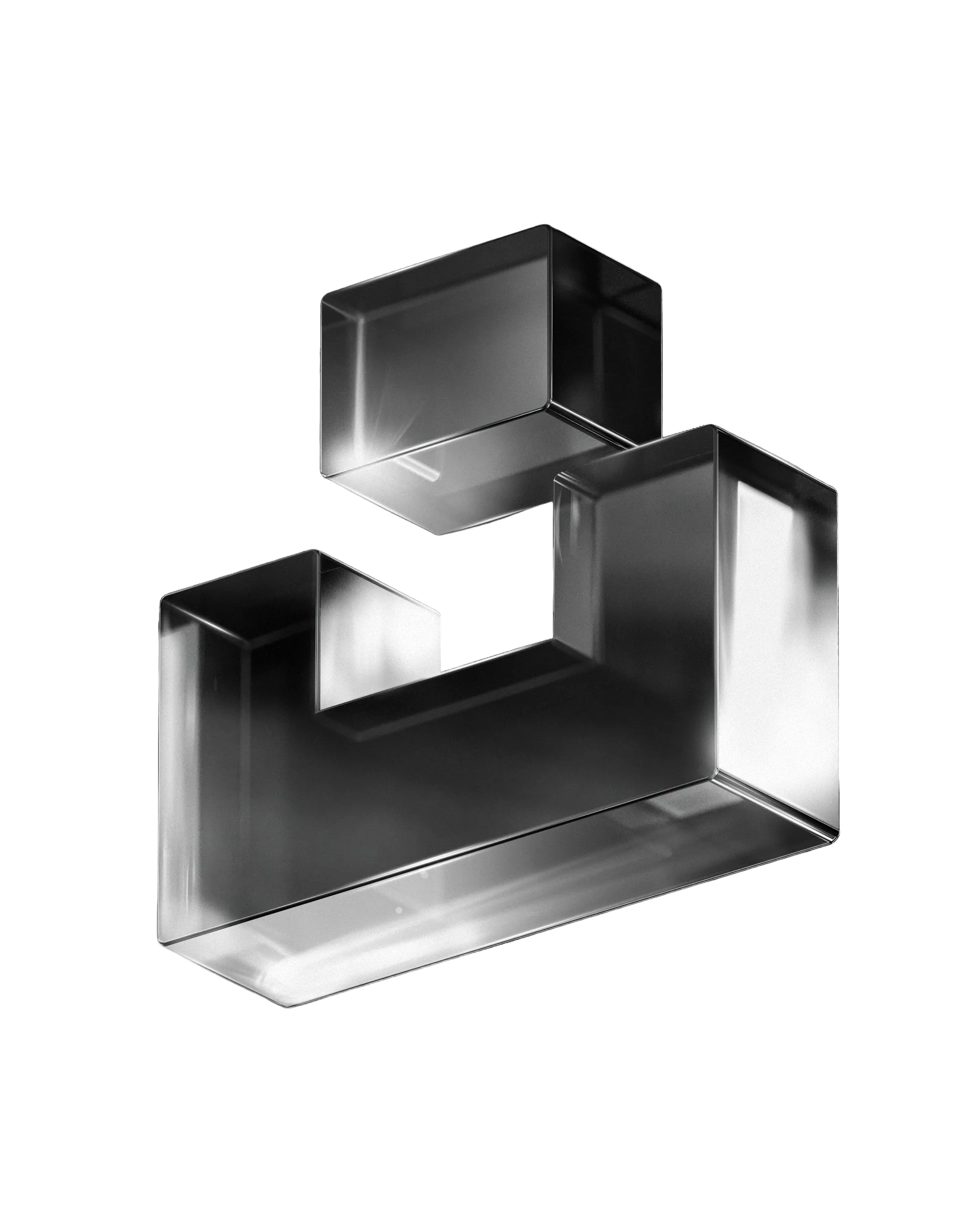
Noti
Documentation
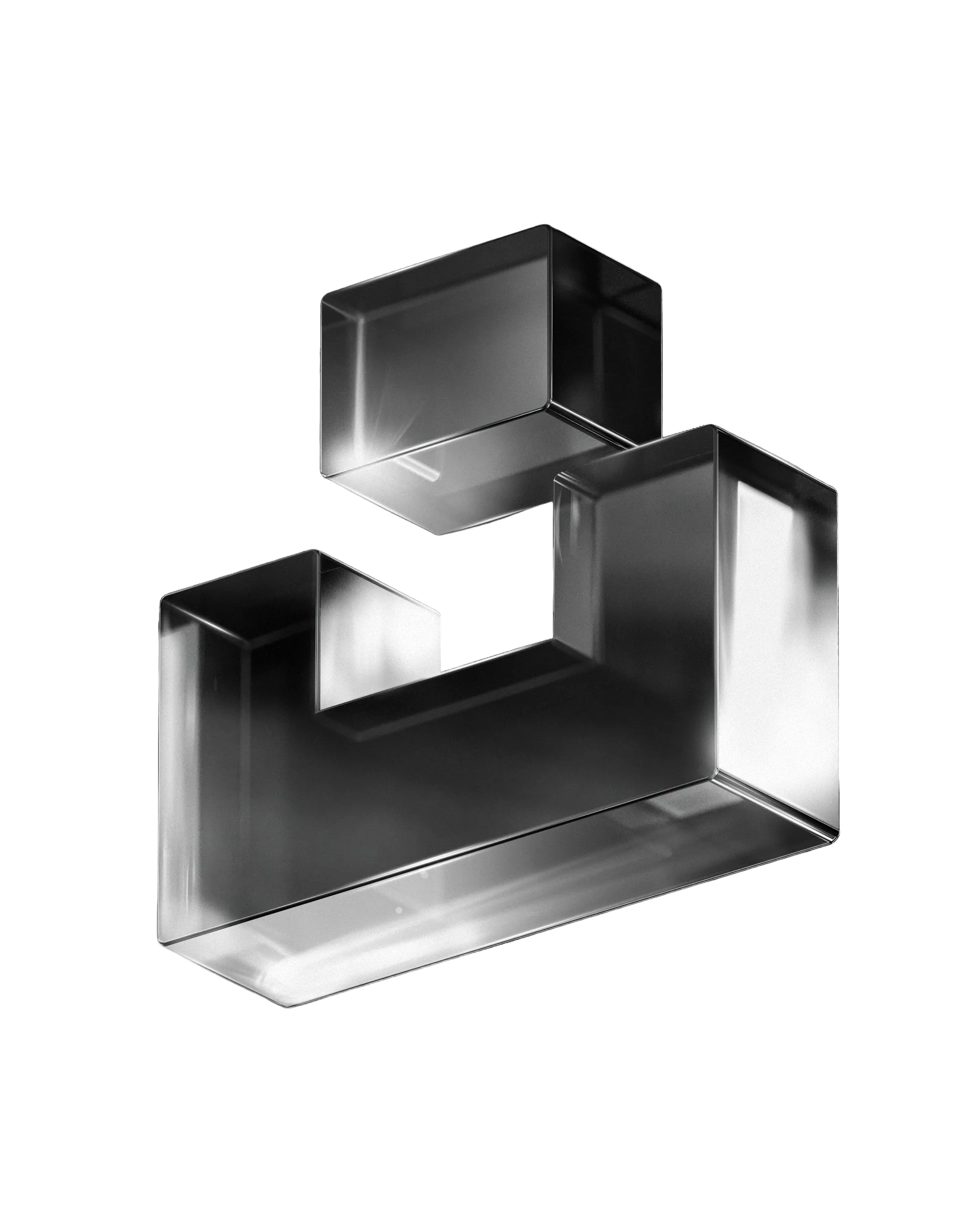
Noti
Documentation
API Reference
Coming Soon with ChatGPT Integration
The full API reference documentation will be available alongside our upcoming ChatGPT integration.
Currently, the Noti Canvas MCP Server works exclusively with Claude Desktop through the Model Context
Protocol.
When available, this section will contain detailed information about all Noti API endpoints for integrating Canvas
data into custom applications, ChatGPT custom actions, and other AI workflows.
Authentication
The upcoming API will use secure authentication methods to protect your Canvas data while enabling
seamless integration with AI tools and custom applications.
RESTful API Endpoints
- • Course and content listing
- • File content extraction
- • Smart document search
- • Assignment management
Rate Limiting
While the current MCP server runs locally with no rate limits, the upcoming API will implement
fair usage policies to ensure reliable access for all users.
The upcoming API will enable:
Course Management
Programmatic access to course listings, enrollment data, and content structures.
File Operations
Upload, download, and process academic documents with AI-powered content extraction.
Assignment Integration
Create, submit, and grade assignments through automated workflows and AI assistance.
Discussion Access
Read forum posts, announcements, and participate in academic discussions programmatically.
Content Search
Advanced search capabilities across all course materials using natural language queries.
Document Processing
Extract and analyze content from PDFs, presentations, and other academic file formats.
Get Started Today
Start with the MCP server now, and be ready for the API when it launches.